Troubleshooting Search Issues & Data Encoding : [No Results & Mojibake] Solutions
Why does seemingly innocuous data transform into a cryptic jumble, leaving you staring at a screen filled with indecipherable characters? The issue of "mojibake," or character corruption, is a surprisingly common digital ailment, and understanding its roots is the first step toward a cure.
The digital world thrives on the smooth translation of information, from the simple alphabet to the complex nuances of ideograms. Yet, this harmony is sometimes shattered. Imagine attempting to read a vital document only to find it populated with symbols that make no sense. This frustrating experience is often the result of incorrect character encoding, a process that can turn carefully crafted text into an unintelligible mess. This is particularly relevant when dealing with non-English scripts such as Chinese. The issue can arise anywhere data is stored, transmitted, or displayed.
The core of the problem lies in the way computers interpret and represent characters. Characters are stored as numerical codes, which are then converted into their visual representation on a screen. Different character encoding schemes, like UTF-8, GBK, or Shift-JIS, assign different numerical codes to the same character. When a system reads data encoded in one scheme but interprets it using another, the result is mojibake. This can manifest as question marks, boxes, or random characters where the original text should be.
To illustrate, consider the situation where Chinese characters are stored in a MySQL database. A common predicament is the character data being converted into an unexpected format. Let's explore the mechanics behind this encoding, the potential pitfalls, and how to prevent such data corruption, including the use of SQL queries to set proper character sets.
In practical terms, the most common causes of mojibake include:
- Incorrect database character set configuration. Databases must be set to correctly handle the character set used by the stored data, usually UTF-8 for broad compatibility.
- Mismatched character sets during data import/export. Data exported from one system and imported into another might not be interpreted using the correct character set.
- Inaccurate character set declaration. When data is displayed, the system viewing the data might not have the proper character set declaration, leading to incorrect display.
- Errors during data transmission. Transmission protocols can sometimes inadvertently alter the character set, especially when dealing with older systems.
Let's assume the database, table, and column are not set up to handle Chinese characters properly, the characters might be stored in an incompatible encoding and, thus, displayed as mojibake.
The impact of mojibake extends beyond mere aesthetic inconvenience. It can render vital information inaccessible, frustrate users, and erode trust in the digital systems that power modern life. In businesses, this could hinder operations, while in research, it could undermine data integrity.
Often the answer to this issue lies in proper character set declaration and handling.
The question of which encoding is applied to Chinese characters when they are stored in a MySQL database is central to this discussion. Let's delve into a common scenario, for example, a situation where a user intends to store Chinese characters but confronts an encoding issue. The core idea is to identify the correct encoding and configure the database accordingly. Typically, the user needs to:
- Select the right character set: UTF-8 is a standard for representing almost all characters.
- Specify the collation: A collation defines how characters are compared and sorted (e.g., utf8mb4_unicode_ci for case-insensitive comparisons).
- Set up the database, table, and columns: Specify the character set and collation for the database, table, and the column where the Chinese text is stored.
- Import data correctly: Ensure your import tool uses the matching character set.
- Display correctly: Ensure that the display application also uses the same character set and collation to prevent further corruption.
Consider this illustration using Python. The following lines provide a simplified view of the encoding problem. This example shows how, using incorrect encoding, text can be garbled. This is also a clear demonstration of how data corruption works.
# Suppose a string with Chinese charactersoriginal_text ="" # Hello world in Chinese# Try to encode it with a wrong encodingtry: encoded_text = original_text.encode('latin-1') print(f"Encoded (incorrectly): {encoded_text}") # When we try to decode using the correct encoding decoded_text = encoded_text.decode('utf-8', errors='replace') print(f"Decoded (after error): {decoded_text}")except UnicodeEncodeError as e: print(f"Encoding error: {e}")
This Python snippet demonstrates how incorrect character encoding can lead to data corruption. If you attempt to encode Chinese characters using 'latin-1' which does not support those characters, you will face an error. Even if you can encode (although it might be a strange encoding that garbles your text), when you decode it back using 'utf-8' with error handling, you might see some replacements or errors, the original text will no longer appear as intended.
The situation that you face involves an "eightfold/octuple mojibake case". This indicates a high degree of corruption, where the character encoding has been misapplied multiple times, potentially compounding the problem. Recovering from such a case requires careful analysis and potentially multiple rounds of correction. Data might have been stored or interpreted using multiple, incorrect character sets, turning even a simple string into an unrecognizable sequence of symbols.
The solutions typically involve identifying the correct original encoding, performing a series of conversions, and, if necessary, data repair strategies. This can require more advanced techniques than a single character set change. Tools for byte-by-byte analysis and specialized data recovery software can sometimes be needed. In some instances, you may have to reverse multiple transformations.
Below, you will find examples of SQL queries, offering ready-made solutions for addressing the most common mojibake issues, particularly those relating to character encoding in MySQL databases. These queries will help you correct character encoding and prevent future issues.
A vital aspect of preventing and resolving mojibake involves employing suitable SQL queries. These queries, when executed on your database, enable you to adjust character sets, ensuring that your data is consistently stored, retrieved, and displayed correctly. Here is a collection of SQL queries tailored to common problems. Remember to apply them with caution, and to test their effect within a development or test environment before applying them to your production database.
Here are some essential SQL queries that can help manage character encoding in a MySQL database and correct mojibake issues:
1. To change the character set and collation of a database:
ALTER DATABASE your_database_name CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci;
Replace your_database_name
with the actual name of your database. UTF-8 with the appropriate collation should usually be your choice.
2. To change the character set and collation of a table:
ALTER TABLE your_table_name CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci;
Replace your_table_name
with the table name.
3. To change the character set and collation of a specific column:
ALTER TABLE your_table_name MODIFY your_column_name VARCHAR(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci;
Replace your_table_name
, your_column_name
, and specify the data type (e.g., VARCHAR) and size for the column.
4. Checking Database, Table and Column Character Sets: Before making changes, its useful to confirm existing settings.
-- For the databaseSELECT DEFAULT_CHARACTER_SET_NAME, DEFAULT_COLLATION_NAME FROM information_schema.SCHEMATA WHERE SCHEMA_NAME = 'your_database_name';-- For a tableSHOW CREATE TABLE your_table_name;-- For a columnSHOW FULL COLUMNS FROM your_table_name;
5. Converting data from an incorrectly encoded column: Sometimes, even if your column is configured correctly, the data itself is mis-encoded.
-- Convert from the incorrect encoding to UTF-8 (assuming it's currently latin1). Replace the source encoding.UPDATE your_table_nameSET your_column_name = CONVERT(CONVERT(your_column_name USING latin1) USING utf8mb4)WHERE your_column_name LIKE '%?%'; -- Optional: Add a WHERE clause to target potentially affected rows.
Replace latin1
with the incorrect encoding you suspect. If you have double mojibake, try applying the conversion query multiple times, correcting the most recent encoding first. Consider using utf8
or gbk
depending on the initial incorrect encoding.
6. Troubleshooting: If your data is still corrupted, examine the data in the table to check the encoding applied.
SELECT your_column_name, HEX(your_column_name) FROM your_table_name;
The HEX() function shows the hexadecimal representation of each character, allowing you to identify encoding patterns.
7. Important notes on data types: Be aware that, for the utf8mb4 character set, you'll typically need to use a data type that supports it. For strings, this is typically VARCHAR. Ensure your columns, especially those with a higher character count, use a supported type.
8. Backups are vital: Before implementing any of these queries, back up your database to ensure you can revert to the original data if there are issues. Test in a staging environment first.
9. Collation matters: While UTF-8 is a character set, a collation is a set of rules that determines how characters are compared and sorted. Choose a collation, such as utf8mb4_unicode_ci
(case-insensitive, general-purpose), that suits your needs.
10. Data Import/Export and Application of Correct Encoding: When importing data, the import tool must know the character set to correctly interpret the source data. Similarly, when exporting, you should specify the character set to use. The character set applied to your application (e.g., PHP) when retrieving data from the database is critical. Make sure it is consistent with your database's settings.
11. Regular Audits: Conduct periodic audits of database settings and data character sets to prevent and address any potential issues early on.
By following these steps, users can store, retrieve, and display Chinese characters and other international scripts correctly within a MySQL database.
Beyond these basic scenarios, it's important to understand that character encoding issues can also stem from incorrect configurations in other parts of the data pipeline, such as the web server, application code, or the user's browser. This can occur with a lack of consistent handling of character encodings at every stage. It's essential to set the correct HTTP headers.
You might also encounter issues related to the user interface, such as incorrect rendering of the characters on a web page. The following steps help address the user interface:
- HTML Meta Tag: The tag in your HTML document specifies the character set for the page.
- HTTP Headers: The web server should send the correct Content-Type HTTP header. For example, in Apache, this can be set in the .htaccess file.
Header set Content-Type "text/html; charset=utf-8" - PHP Configuration: When creating a PHP web page, it's important to set the correct character set.
Understanding these character set issues helps in finding a solution when users come across mojibake. It might involve the examination of character codes, and the database setup along with the application settings.
The following is a simple chart to illustrate common issues and provide a starting point for troubleshooting:
Chart: Troubleshooting Character Encoding Problems
Problem Scenario | Possible Causes | Suggested Solutions |
---|---|---|
Chinese characters appear as question marks (?) or boxes |
|
|
Garbled characters, e.g., "" instead of "" |
|
|
Double mojibake multiple layers of corruption |
|
|
In conclusion, addressing the issue of character encoding involves setting up a proper database, table, and column. Furthermore, users must be consistent in their character encoding settings across all parts of the data pipeline, including the web server, application code, and the user's browser. The use of appropriate SQL queries offers quick, workable solutions to many problems.

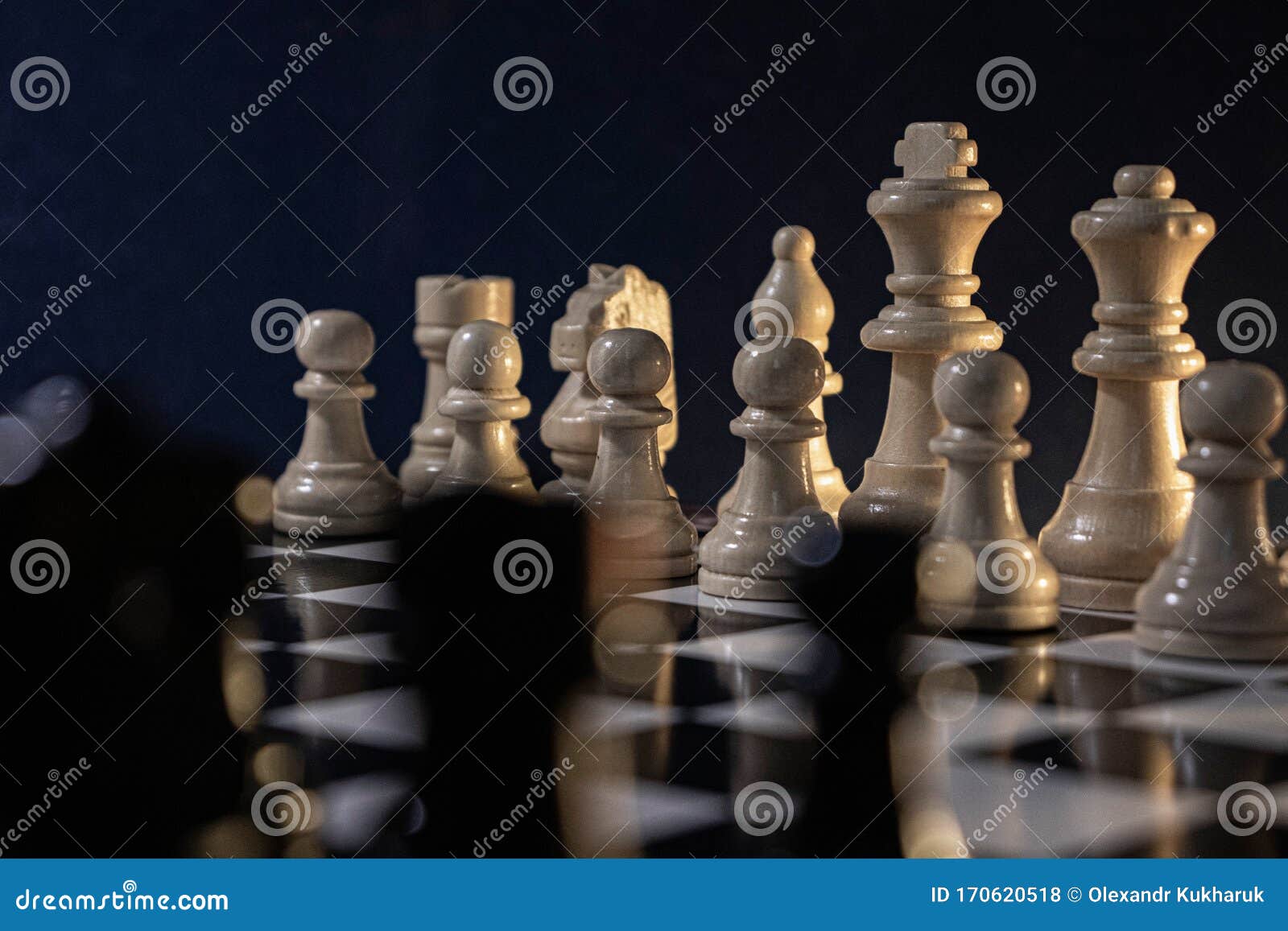
